NestJS is a popular framework for building scalable and maintainable server-side applications using Node.js. In this guide, we will cover the basics of NestJS and help you get started with building your first NestJS application.
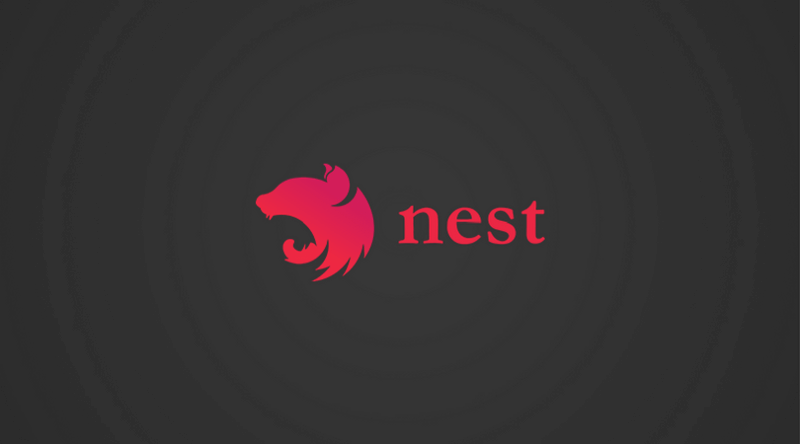
What is NestJS?
NestJS is a framework for building server-side applications using Node.js. It is built on top of Express.js and provides a set of tools and features to help developers build scalable and maintainable applications. NestJS uses a modular architecture and supports a wide range of plugins and modules that can be easily integrated into your project.
Installing NestJS
To install NestJS, you need to have Node.js and npm installed on your system. You can install NestJS using the following command:
npm install -g @nestjs/cli
This will install the NestJS CLI globally on your system, allowing you to create new NestJS projects and run commands from the terminal.
Creating a New NestJS Project
To create a new NestJS project, you can use the following command:
nest new my-app
This will create a new NestJS project in a directory named my-app
. You can change the name of the project by replacing my-app
with a different name.
Running the NestJS Application
To run the NestJS application, navigate to the project directory and use the following command:
npm run start
This will start the NestJS application and make it available on http://localhost:3000
.
Creating a Basic NestJS Controller
A controller in NestJS is responsible for handling incoming requests and returning responses. To create a basic controller, you can use the following command:
nest generate controller cats
This will generate a new controller named cats
in your project directory. The cats.controller.ts
file will contain a basic template for a controller.
import { Controller, Get } from '@nestjs/common'; @Controller('cats') export class CatsController { @Get() findAll(): string { return 'This action returns all cats'; } }
In this example, we have created a CatsController
that handles incoming requests to the /cats
endpoint. The findAll
method returns a simple string response.
Creating a Basic NestJS Service
A service in NestJS is responsible for implementing business logic and interacting with data sources. To create a basic service, you can use the following command:
nest generate service cats
This will generate a new service named cats
in your project directory. The cats.service.ts
file will contain a basic template for a service.
import { Injectable } from '@nestjs/common'; @Injectable() export class CatsService { private readonly cats: string[] = ['Garfield', 'Tom', 'Sylvester']; findAll(): string[] { return this.cats; } }
In this example, we have created a CatsService
that returns a list of cats. The cats
array is defined in the service’s constructor and is returned by the findAll
method.
Using the NestJS Controller and Service Together
To use the controller and service together, we need to inject the CatsService
into the CatsController
. We can do this by adding a constructor to the CatsController
and injecting the service using the @Inject
decorator.
import { Controller, Get, Inject } from '@nestjs/common'; import { CatsService } from './cats.service'; @Controller('cats') export class CatsController { constructor(private readonly catsService: CatsService) {} @Get() findAll(): string[] { return this.catsService.findAll(); } }
In this example, we have added a constructor to the CatsController
and injected the CatsService
using the @Inject
decorator. The findAll
method now calls the findAll
method on the CatsService
to return a list of cats.
Conclusion
In this guide, we covered the basics of NestJS and helped you get started with building your first NestJS application. We showed you how to install NestJS, create a new project, and run the application. We also created a basic controller and service, and showed you how to use them together.
NestJS provides a powerful set of tools and features to help you build scalable and maintainable server-side applications. With NestJS, you can take advantage of the best practices and patterns from other popular frameworks, while still using the flexibility and power of Node.js. We hope this guide has been helpful, and we wish you the best of luck with your NestJS development!