Augmented Reality (AR) is a captivating technology that fuses the digital and physical worlds, offering countless possibilities for interactive experiences. In this tutorial, Codevivu will explore how to implement an AR camera using AR.js and Babylon.js, two powerful JavaScript libraries. By the end of this guide, you will have the knowledge and code to create your own AR camera application.
Prerequisites
Before diving into AR.js and Babylon.js, make sure you have the following:
- Basic knowledge of HTML, JavaScript, and 3D graphics concepts.
- A text editor for writing code (e.g., Visual Studio Code).
- A modern web browser with support for WebRTC and WebGL (e.g., Chrome or Firefox).
- A stable internet connection.
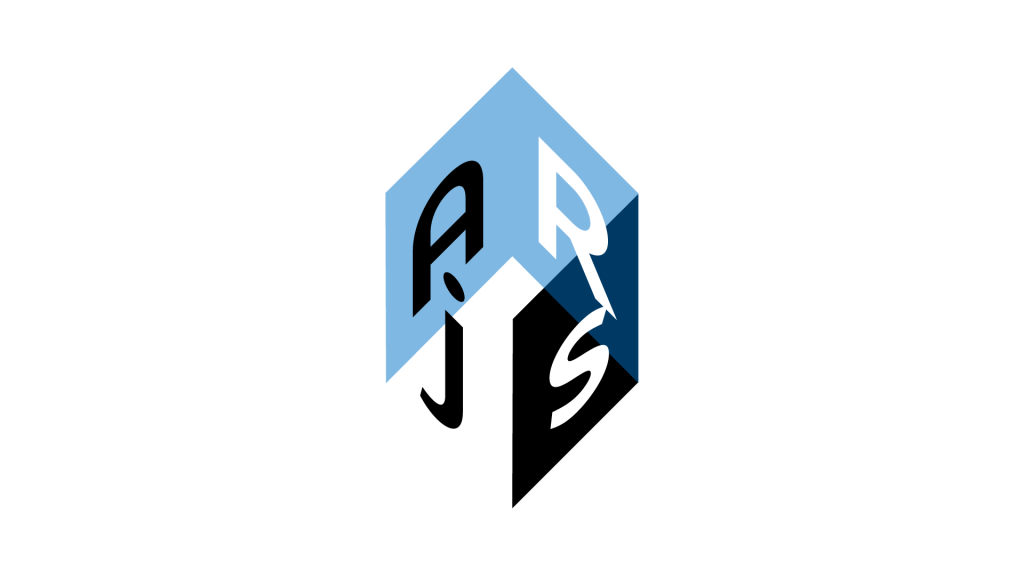
Step 1: Setting Up Your Development Environment
To get started with AR.js and Babylon.js, follow these steps:
- Create a new project directory on your computer.
- Inside the directory, create an HTML file (e.g.,
index.html
) and a JavaScript file (e.g.,app.js
). - Download AR.js and Babylon.js from their respective official websites and include them in your project directory.
Step 2: Creating the HTML Structure
In your index.html
file, set up the basic HTML structure and include the necessary scripts and stylesheets:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AR Camera with AR.js and Babylon.js</title>
<script src="path/to/babylon.js"></script>
<script src="path/to/ar.js"></script>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
Replace "path/to/babylon.js"
and "path/to/ar.js"
with the actual paths to the downloaded Babylon.js and AR.js libraries.
Step 3: Setting Up the AR Scene
Now, let’s create the AR scene in app.js
:
// Initialize AR.js
ARController.getUserMediaThreeScene({
onSuccess: function (arScene, arController, arCamera) {
document.body.appendChild(arScene.canvas);
// Initialize Babylon.js scene
var engine = new BABYLON.Engine(arScene.canvas, true);
var scene = new BABYLON.Scene(engine);
// Babylon.js camera
var camera = new BABYLON.ArcRotateCamera("camera", 0, 0, 0, BABYLON.Vector3.Zero(), scene);
camera.attachControl(arScene.canvas, true);
// Babylon.js light
var light = new BABYLON.HemisphericLight("light", new BABYLON.Vector3(0, 1, 0), scene);
// Load and add a 3D model
BABYLON.SceneLoader.ImportMesh("", "path/to/", "your-3d-model.glb", scene, function (meshes) {
// Set the model's position and scale
meshes[0].position.set(0, 0, 0);
meshes[0].scaling.set(0.1, 0.1, 0.1);
});
// Babylon.js render loop
engine.runRenderLoop(function () {
scene.render();
});
},
onError: function () {
console.error("Error accessing the camera.");
}
});
Replace "path/to/your-3d-model.glb"
with the actual path to your 3D model file.
Step 4: Testing Your AR Camera
Save your changes and open the index.html
file in a modern web browser that supports AR.js and Babylon.js. Allow camera access when prompted, and you should see your AR camera overlaying the 3D model on the marker.
Congratulations! You’ve successfully implemented an AR camera using AR.js and Babylon.js. This combination allows you to create immersive AR experiences that blend the real world with 3D content seamlessly. Explore more features and capabilities of both libraries to enhance your AR projects further.
Conclusion
AR.js and Babylon.js are powerful tools for building AR applications on the web. In this tutorial, we’ve covered the basics of setting up an AR camera, adding markers, and displaying 3D content using these libraries. Feel free to experiment and expand upon this foundation to create your own unique and captivating AR experiences. Happy coding!